Cracking Passwords using Hashcat
In the infosec, password cracking is considered as the easiest part given that the encrypted password exists in the wordlist and you are patient enough to wait. Learn basics of the hashcat password cracking tool and how to prevent from being the target of password attack
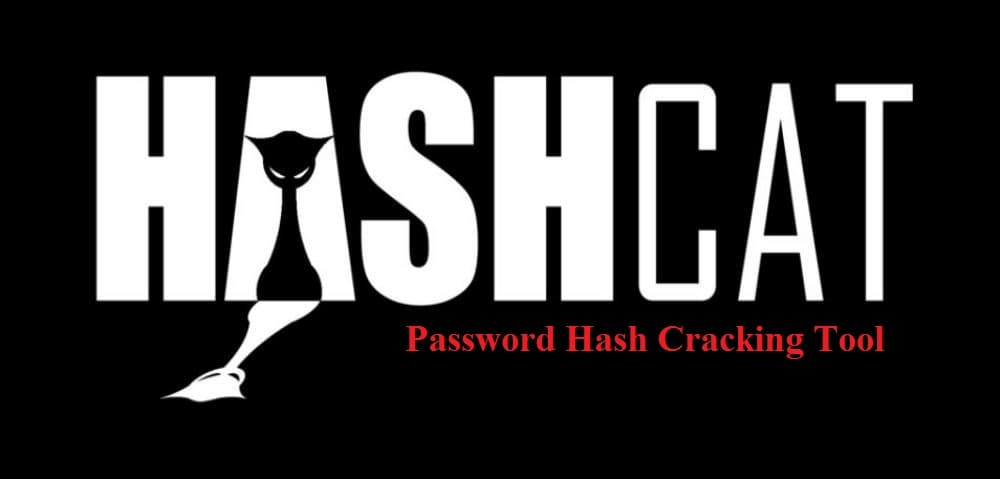
While pentesting, most of the time you will find encrypted passwords stored in DB that you might have accessed by exploiting some SQL vulnerability or DB config file recovery. It would be very rare that the developer has configured to store the plain text password. What you will do in that case, give up / ignore it? No way! That is not an option here. You can use the password cracking tools to find the password.
What is Hashing?
Hashing is the process of generating a string of fixed length based on the input data provided to a hashing function of one type. The hashes are often used as a checksum to ensure that the file is legit and is not tampered with in between the transmission. Once a password is hashed via any hashing algorithm, let's say md5 here. You can not retrieve the original text from it as the information and rounds of encrypting is known to you. Such types of hash algorithms are called One-Way Hashing Algorithm. The most common hashing functions are Sha-256, MD5, Sha-1 and Sha-512.
To find the password you can either get a wordlist containing common passwords or a brute-forcing charset. Pass the input to the hash function and compare it with the hash you want to crack. If it matches, you cracked the password. The following is intuition on how the password cracking function would look like.
import sys, hashlib
with open(sys.argv[2], "rb") as hash_file:
for hash in hash_file:
hash = hash.strip().decode()
with open(sys.argv[1], "rb") as wordlist_file:
for line in wordlist_file:
line = line.strip()
digest = hashlib.md5(line).hexdigest()
if hash == digest:
print(hash, line.decode(), sep=":")
break
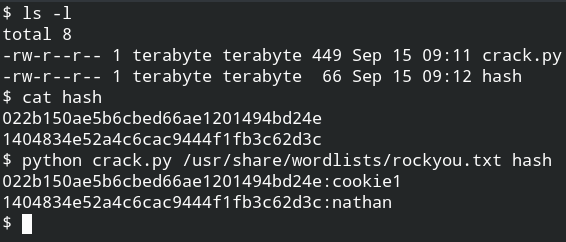
NOTE – Do not confuse hashing with encoding. In the case of encoding, there is also a decoding function that will help you convert the ciphered text back to its original form. It can or can not be secured by the keys. For example – Base64, DES, AES, etc...
What is Hashcat and Why use it?
The python script you have seen works well on small wordlists but will take forever for a gigantic set of passwords and will be extremely slow (even if you implement multiprocessing). Hashcat is a blazingly fast solution to the problem we have with high-level languages. It is written in C language and provided kernel modes to execute the hash function directly on the GPU (100x CPU power) to calculate the compare the hash string.
It supports almost all the algorithms and their combination with/without salt. This software is free to use and is open-source licensed under MIT license.
Website – https://hashcat.net/hashcat/
Forum – https://hashcat.net/forum/
GitHub Repository – https://github.com/hashcat/hashcat
Hachcat in Action
In this section, I will demonstrate how to use hashcat and launch a wordlist attack on the list of passwords stored in the hash file. You can practice this lab on AttackDefense.
You have two files for the wordlist and the hash digest. The digest in this case is a bcrypt-md5 hash. On the hashcat wiki page, here, you will have access to all the supported flags and their example. Match the example with digest and you can too find the appropriate hash type.
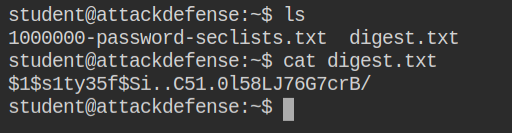
Hashcat doesn't support the name of the hash, you need to provide the numeric id of it while launching the attack. You don't need to memorize these values, they can be found from hashcat help or the example page.
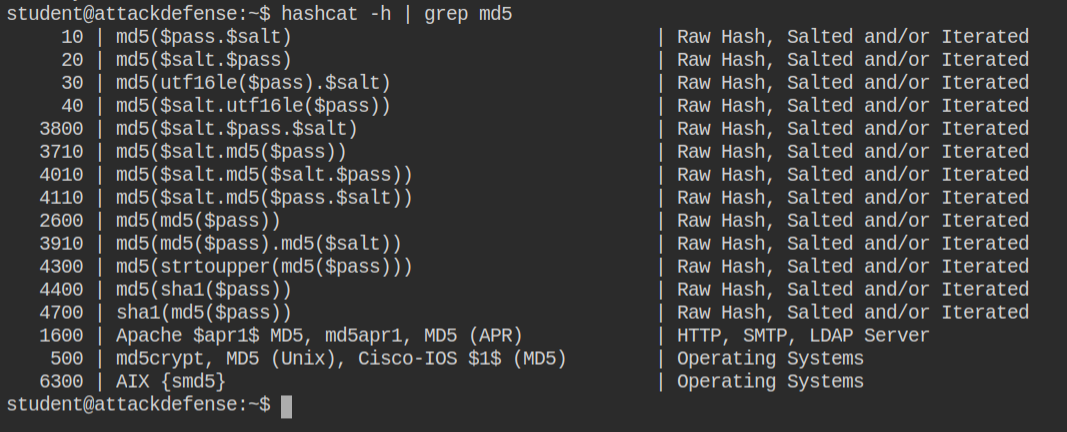
Now you have everything that is required for cracking the password. Simply launch the brute force attack by executing the following command. Here, -m
the option will take the value of the hash and -a
will take the attack mode. Here 0
means use dictionary attack. It will require you to pass the digest file followed by the wordlist file.
hashcat -a 0 -m 500 digest.txt 1000000-password-seclists.txt
Give it few seconds to initialize the kernel and start the comparison. In few milliseconds it will break the password and give you the plain text in format HASH:PlainText. This is because it supports batch processing.
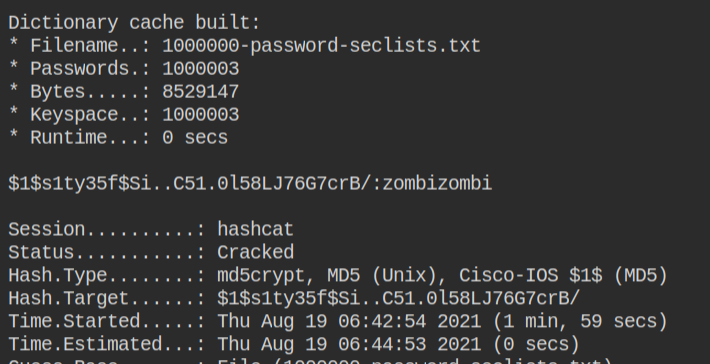
Conclusion
Passwords are a crucial part of infosec and are being used in every application to provide authentication. End users often think that they can choose easily memorable passwords and it's the duty of the developer and the security team to protect their account, which is not the case. They can ensure the security of the infrastructure but not the user input. Anyone who has penetrated the application and dumped the credentials can download the appropriate wordlist and crack the password. Here is an article from the Times of India which tells that 80% of attacks are linked with bad passwords. To ensure your account is safe you need to do the following
- Don't use common passwords like jerry1234, cookie123, mypass@123
- Don't use embarrassing passwords that tell about your mood and personality, like I@mtheB055, Ih@t3MyJ0b, myB055Sucks, etc
- Don't repeat your password across multiple sites, because if one site is compromised attackers can try the same password on different applications. This technique is known as Password Reuse Vulnerability
- Use tools like https://passwords-generator.org/ to ensure the password is strong and difficult to crack. If you are looking for an advanced tool that can tell you how long it will take to bruteforce your password or whether it is being leaked in any hack till now, I would recommend you https://www.experte.com/password-generator.
- Frequently change your password so that even if it is cracked and it becomes ineffective when the attacker tries to log in with an old password
- Never leave any hint or store the password at the place where anyone can reach like saving in a third-party password managers